In this chapter we shall learn about below topics:
4.1 Introduction to Double Linked List
4.2 Representation of Double Linked List
4.3 Operations performed on Double Linked List
4.4 Implementation of Single Linked List
4.5 Output of the program
In the previous chapter we learnt about single linked list. In this chapter we shall learn about Doubly Linked List.
Doubly Linked List is a special data structure, which is a collection of zero or more nodes.
4.1 Introduction to Double Linked List
Each node is made up of 3 parts, “prev_link + data + next_link”. As it is a Doubly linked list, we need to store the address information for the previous node and the next node.
prev_link: It is used to hold the information of the previous node.
Data: It is used to store some value. It can be an Integer variable or a structure.
next_link: It is used to hold the information of the next node.
Together with “prev_link + data + next_link” is called as a node.
4.2 Below is how we represent a doubly LL node:
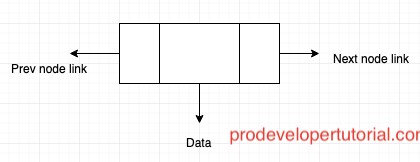
A DLL with multiple nodes can be shown below:
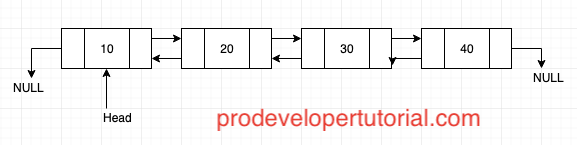
From the above image we can infer following below points:
1. First node prev_link will always be null
2. Last node next_link will always be null
3. Data section holds the data
4. Head pointer always point to the first node
4.3 Below are the operations that we will be learning in DLL
1. Insert element in rear.
2. Delete element with given key value.
3. Search an element.
4. Display all the data.
1. Insert element in rear.
While inserting element at the rear, we need to check 2 conditions.
1. If the head node is NULL
If the head node is NULL, it means that the element we are trying to insert is the first element.
Hence we do below steps:
1. Copy the value to the data section
2. Make prev_link and next_link pointers point to NULL.
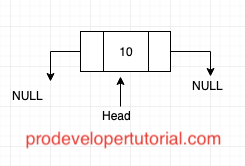
2. If the head node is not NULL
If the head element is not NULL, it means that there are already elements present. Hence we do the following steps to insert at the rear.
1. Take a new node and copy the value.
2. Take a temp pointer, traverse till the end of the list.
3. Make the prev pointer of new node to point to temp node
4. Make the next pointer of the new node to point to NULL.
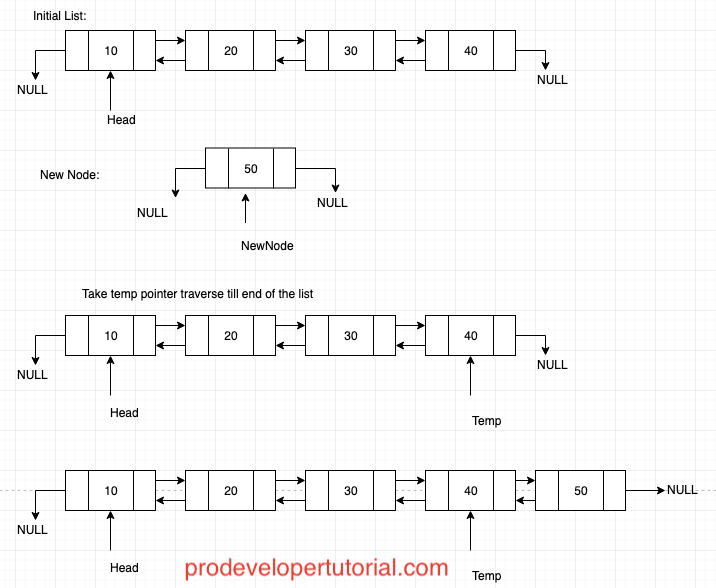
2. Delete element with given key value.
To delete we follow below steps:
1. Take a temp pointer move to the point till you find the element equal to the key.
2. If the element is the lest element, then make the previous element’s next pointer to NULL.
3. Else, do the following operations:
1. temp->prev->next = temp->next;
2. temp->next->prev = temp->prev;
4. Delete temp node.
3. Search an element.
To search an element, we do below steps:
1. Take a temp pointer pointing to head
2. Check if the data is same as key
3. If it is not, move the temp pointer to next node
4. Do step 2 and 3 till you find the key.
4. Display all the data.
To Display, we do below steps:
1. Take a temp pointer pointing to head
2. Display the data
3. Move the temp pointer to next node
4. Do step 2 and 3 till you reach the end of LL.
4.4 Implementation of Single Linked List
#include<iostream>
using namespace std;
struct Node
{
int val;
Node *prev;
Node *next;
};
Node *head;
void insert_rear(int value)
{
Node *temp = head;
if (head != NULL)
{
// if head is not null
while(temp->next != NULL)
{
temp = temp ->next;
}
Node *newNode = new Node;
temp->next = newNode;
newNode->prev = temp;
newNode->val = value;
newNode->next = NULL;
}
else
{
//if head is nul
Node *newNode = new Node;
newNode->val = value;
newNode->prev = NULL;
newNode->next = NULL;
head = newNode;
}
}
void remove(int x)
{
Node *temp = head;
while(temp->val != x)
{
temp = temp->next;
}
if(temp -> next == NULL)
{
temp->prev->next = NULL;
}
else
{
temp->prev->next = temp->next;
temp->next->prev = temp->prev;
}
delete temp;
}
void search(int x)
{
Node *temp = head;
int found = 0;
while(temp != NULL)
{
if(temp->val == x)
{
cout<<"\nFound";
found = 1;
break;
}
temp = temp->next;
}
if(found==0)
{
cout<<"\nNot Found";
}
}
void display()
{
Node *temp =head;
while(temp !=NULL)
{
cout<< temp->val<<"\t";
temp = temp->next;
}
}
int main()
{
int choice, x;
do
{
cout<<"\n1. Insert";
cout<<"\n2. Delete";
cout<<"\n3. Search";
cout<<"\n4. Display";
cout<<"\n5. Exit";
cout<<"\n\nEnter you choice : ";
cin>>choice;
switch (choice)
{
case 1 : cout<<"\nEnter the element to be inserted at rear : ";
cin>>x;;
insert_rear(x);
break;
case 2 : cout<<"\nEnter the element to be removed : ";
cin>>x;
remove(x);
break;
case 3 : cout<<"\nEnter the element to be searched : ";
cin>>x;
search(x);
break;
case 4 : display();
break;
}
}
while(choice != 5);
return 0;
}
4.5 Output of the program
1. Insert
2. Delete
3. Search
4. Display
5. Exit
Enter you choice : 1
Enter the element to be inserted at rear : 1
1. Insert
2. Delete
3. Search
4. Display
5. Exit
Enter you choice : 1
Enter the element to be inserted at rear : 2
1. Insert
2. Delete
3. Search
4. Display
5. Exit
Enter you choice : 1
Enter the element to be inserted at rear : 3
1. Insert
2. Delete
3. Search
4. Display
5. Exit
Enter you choice : 1
Enter the element to be inserted at rear : 4
1. Insert
2. Delete
3. Search
4. Display
5. Exit
Enter you choice : 4
1 2 3 4
1. Insert
2. Delete
3. Search
4. Display
5. Exit
Enter you choice : 3
Enter the element to be searched : 3
Found
1. Insert
2. Delete
3. Search
4. Display
5. Exit
Enter you choice : 2
Enter the element to be removed : 3
1. Insert
2. Delete
3. Search
4. Display
5. Exit
Enter you choice : 4
1 2 4
1. Insert
2. Delete
3. Search
4. Display
5. Exit
Enter you choice : 5