In this tutorial we shall see how to print level by level of the tree nodes.
Problem Statement:
Given the root node of the tree, print the nodes level by level.
For example:
Consider the tree below:
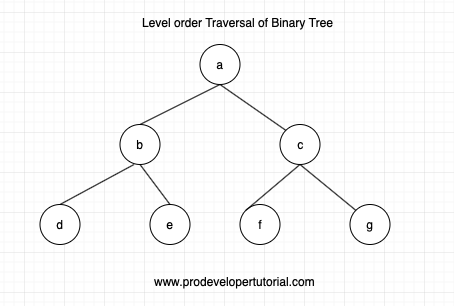
Here the level order traversal will be as below:
[a]
[b, c]
[d, e, f, g]
i.e we need to print the nodes level by level.
Level order traversal can also be written as below:
a, b, c, d, e, f, g
i.e without printing level by level, but by printing in single line.
We can solve this problem with help of queues.
Basic Idea:
Step 1: Enqueue the node
Step 2: Dequeue the node and print
Step 3: Enqueue left and right child of the node.
Now let’s apply these steps and check our result.
First enqueue the root node
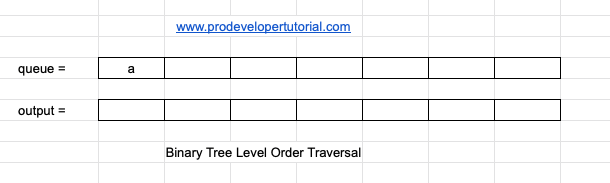
Now move the node “a” to output, mark it as visited. Insert left and right child of node “a”.
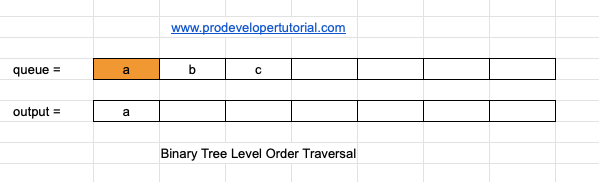
Now select node ”b” move it to output. Then insert it’s children in queue.
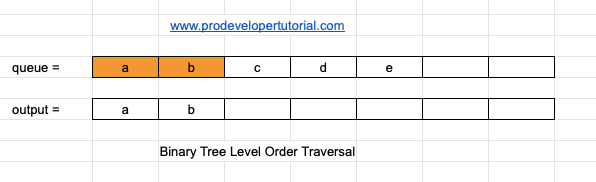
Now select node “c” move it to output. Insert it’s left and right children in to the queue.
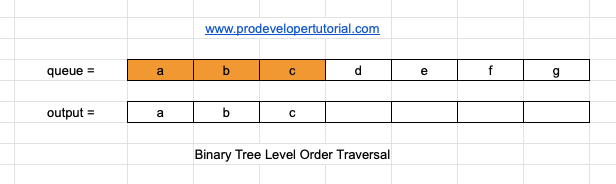
Similarly, if we do the same operation, we get our result.
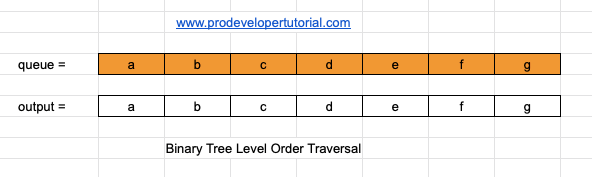
Now let’s see how to print level by level:
We follow below steps:
Step 1: Enqueue root
Step 2: Enqueue Null
Step 3: dequeue node and null
Step 4: Enqueue left and right child
Step 5: If the element is null then,
a. Go to the next line
b. Enqueue null.
Now let’s see the above steps by taking an example:
Initially we insert root node “a” and null.
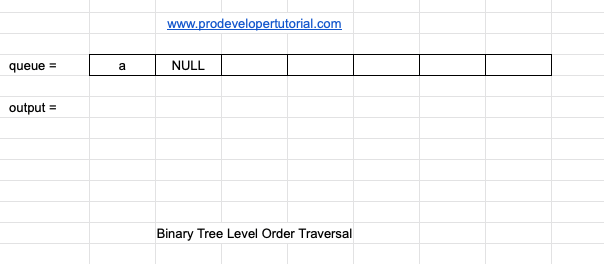
Now, dequeue a into the output vector.
Insert it’s left and right child as shown, mark it as visited.
Note that till now we are in the first line.
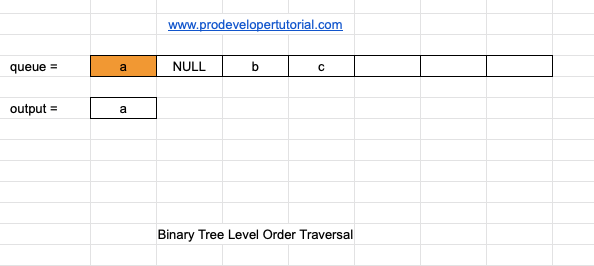
Now, dequeue NULL, so when we dequeue null, we go to the next line and also insert NULL in to the array.
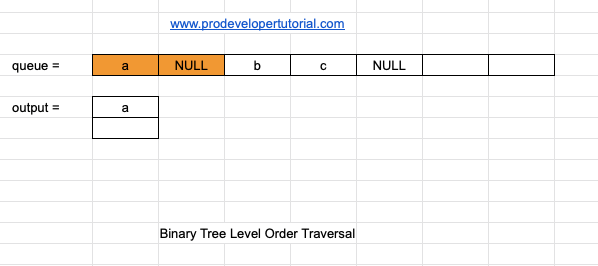
Now in the next 2 iterations, we print b, insert its children d, e and print c, and insert it’s children f, g.
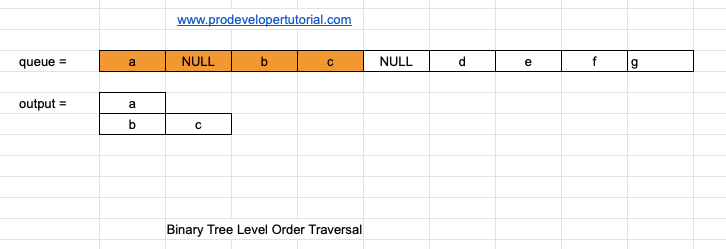
Now we reach NULL, we go to the next line and insert NULL into the queue.
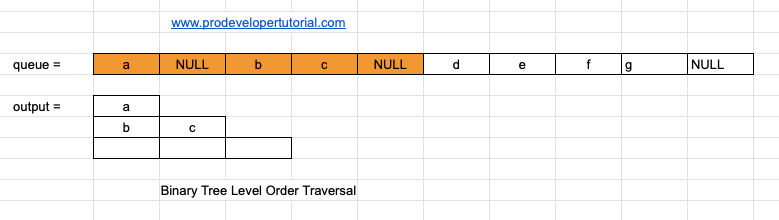
In the end we get the result as below:
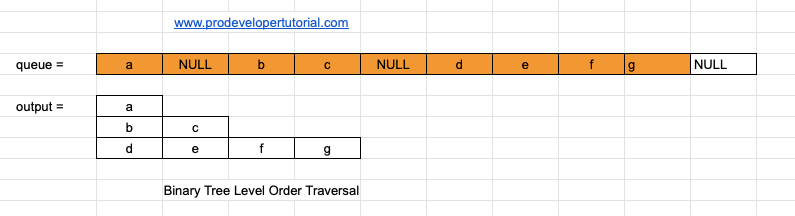