In this chapter we shall have a look at:
8.1. Heaps introduction
8.2. Different types of heaps
8.3. Operations that can be performed on heaps
8.4. C++ program to implement heaps
8.1 Heaps introduction:
Heap is a tree based data structure that satisfies a property. This property varies on the type of heap used.
What is that property? We shall have a look at it below:
8.2 Heaps can be divided into 2 types.
Based on the type the property will differ. The 2 types of heap are:
1. Min heap
2. Max Heap
1. Min heap:
In minimum heap, the value of the parent node will always be less than or equal to their child node in entire tree.
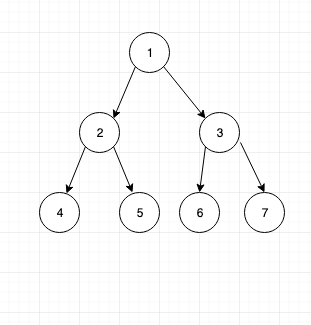
2. Max heap:
In this type of heap, the value of parent node will always be greater than or equal to their child node in the entire tree
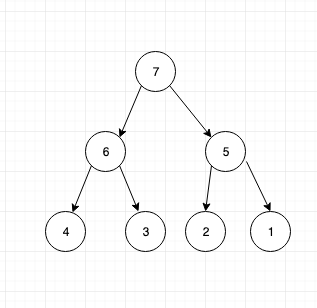
As heap is also a tree, a single parent can have many children. But usually in practice, we see a node [parent node] having at most 2 children. Hence such type of heap is called as “Binary Heap”.
8.3 Below are the operations that are performed on a binary heap:
1. Get Max, Get Min
2. Insert into heap
3. Initialize a heap
4. Heapify : Create a heap, given an array of elements.
Although heaps are tree based algorithm, they are implemented using arrays. C++ standard library provides algorithms to create and manipulate heaps.
Basic formula to get the child index:
As heaps are represented using arrays, at any point we can find the left child and/or right child by using below formula.
Left Child Index = 2 * parent_index + 1
Right Child Index = 2 * parent_index + 2
Similarly, to know the parent index for a child, it can be found by using the formula:
Parent_index = (child_index -1)/2
Now let us see how to insert an element into heap.
Here we shall take max heap as an example.
To insert an element, first we insert it at the bottom/end of the heap data structure.
Then we rebuild heap to place the new element at its correct position.
To rebuild the heap, we follow below steps:
1. Start with the bottom node.
2. Get the parent node.
3. If the child node is greater than the parent node, swap the elements else quit.
Now let us see how to remove max element from the heap:
To remove the element from heap, swap the first and last element of the array/heap.
Decrement the “bottom” of the array, thus removing the element.
Then rebuild the heap/array to restructure the newly formed array by following below steps.
1. Start from the top element, identify it’s children.
a. If it has no children, then it has been heapify. Hence quit.
2. Else, get the greater of the 2 children. If the value of child is greater than parent, swap else quit.
8.4. C++ program to implement heaps
#include<iostream>
using namespace std;
int *ptr_heap_arr; //pointer to the array elements of heap
int max_heap_capacity; // maximum size of min heap as set by user
int current_heap_size; // Current number of elements in min heap
//this function is used to swap 2 integer values
void swap(int *a, int *b)
{
int temp = *a;
*a = *b;
*b = temp;
}
int get_parent(int i)
{
return (i-1)/2;
}
// get left child
int left_child(int i)
{
return (2*i + 1);
}
// get right child
int right_child(int i)
{
return (2*i + 2);
}
// initialize min heap
void min_heap(int cap)
{
current_heap_size = 0;
max_heap_capacity = cap;
ptr_heap_arr = new int[cap];
}
// helper function to heapify a subtree with root at given index
void min_heapify(int i)
{
int l = left_child(i);
int r = right_child(i);
int smallest = i;
if (l < current_heap_size && ptr_heap_arr[l] < ptr_heap_arr[i])
smallest = l;
if (r < current_heap_size && ptr_heap_arr[r] < ptr_heap_arr[smallest])
smallest = r;
if (smallest != i)
{
swap(&ptr_heap_arr[i], &ptr_heap_arr[smallest]);
min_heapify(smallest);
}
}
// function to remove minimum element from min heap
int extractMin()
{
if (current_heap_size <= 0)
return INT_MAX;
if (current_heap_size == 1)
{
current_heap_size--;
return ptr_heap_arr[0];
}
int root = ptr_heap_arr[0];
ptr_heap_arr[0] = ptr_heap_arr[current_heap_size-1];
current_heap_size--;
//heapify the new tree
min_heapify(0);
return root;
}
void insert_element(int k)
{
if (current_heap_size == max_heap_capacity)
{
cout << "\n max heap capacity has been reached";
return;
}
current_heap_size++;
int i = current_heap_size - 1;
ptr_heap_arr[i] = k;
while (i != 0 && ptr_heap_arr[get_parent(i)] > ptr_heap_arr[i])
{
swap(&ptr_heap_arr[i], &ptr_heap_arr[get_parent(i)]);
i = get_parent(i);
}
}
int main()
{
min_heap(11);
insert_element(3);
insert_element(2);
insert_element(14);
insert_element(6);
insert_element(7);
insert_element(35);
cout <<extractMin() << " ";
return 0;
}
8.5. Output
2